Created: Nov 4, 2020 5:23 PM
springboot 특징
- 단독 실행(stand-alone) 가능한 스프링 애플리케이션을 생성한다.
- 내장 서버(web container)를 포함하고 있다.
- 의존성 관리
- 빌드 구성을 단순화하는 starter 제공하여 인코딩,플러그인,버전 등 의존성을 관리해준다
- Auto Configuration
- xml이나 java 설정이 필요 없이 의존성과 환경 변수만 설정하면 된다.
↔ 기존 스프링에서 겪었던 설정의 어려움을 해소 → initializer를 통해 빠르고 쉽게 프로젝트 구성 가능하다.
spring initializr 를 통해 프로젝트 생성
빌드 툴, 언어, 스프링 부트 버전, 프로젝트 메타데이터 설정
사용할 depedencies 등록
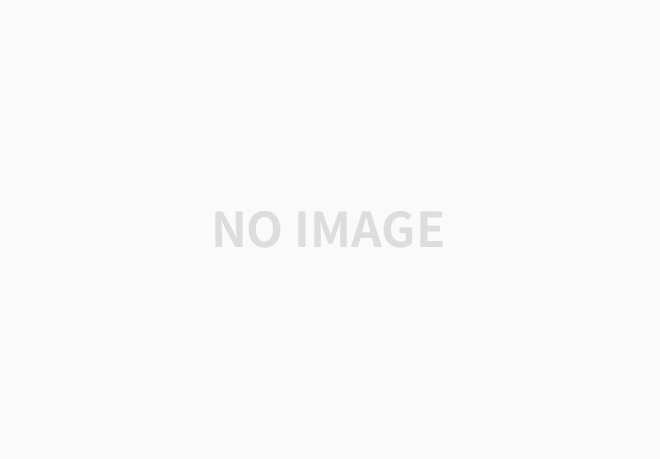
spring boot 의존성 관리
spring-boot-starter를 통해 dependency간 충돌 문제가 없는 버전으로 자동 설정해줌
pom.xml
→ spring-boot-starter-parent-*.*.*.RELEASE.pom
→ spring-boot-dependencies-2.3.5.RELEASE.pom
의 상속 관계를 가짐. spring-boot-dependencies-2.3.5.RELEASE.pom
에 설정되어 있는 버전으로 자동으로 매핑된다.
pom.xml
<!-- pom.xml -->
<!-- Inherit defaults from Spring Boot -->
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.5.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<!-- Add typical dependencies for a web application -->
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
spring-boot-dependencies-2.3.5.RELEASE.pom
<properties>
<commons-dbcp2.version>2.7.0</commons-dbcp2.version>
<hikaricp.version>3.4.5</hikaricp.version>\
...
</properties>
...
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-test</artifactId>
<version>2.3.5.RELEASE</version>
</dependency>
spring boot auto configuration
스프링 부트는 애플리케이션의 설정을 자동으로 해준다.
@SpringBootApplication
은 @ComponentScan
과 @EnableAutoConfiguration
을 포함하고 있다.
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
@EnableAutoConfiguration
은 스프링에서 통상적으로 사용하는 빈들을 자동으로 컨테이너에 등록해주는 어노테이션이다. @EnableAutoConfiguration이 기본으로 등록하는 빈 목록은 spring-boot-autoconfigure-2.X.X.jar 안의 spring.factories파일에 들어있다.
# Auto Configure
org.springframework.boot.autoconfigure.EnableAutoConfiguration=\
org.springframework.boot.autoconfigure.admin.SpringApplicationAdminJmxAutoConfiguration,\
org.springframework.boot.autoconfigure.aop.AopAutoConfiguration,\
org.springframework.boot.autoconfigure.data.jdbc.JdbcRepositoriesAutoConfiguration,\
org.springframework.boot.autoconfigure.data.jpa.JpaRepositoriesAutoConfiguration,\\
** @Enable*
은 특정 기준의 설정 클래스를 로드한다. ~@EnableAutoConfiguration, @EnableWebMvc
이 때, spring.factories의 모든 클래스를 무조건 등록하는 것이 아니라 @Conditional 어노테이션의 조건에 따라 등록한다.
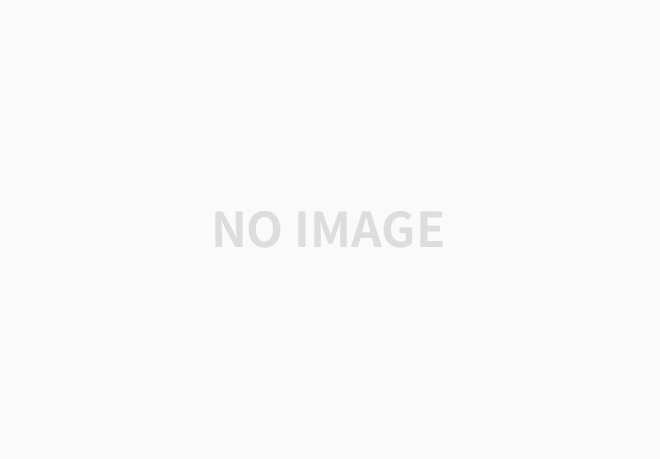
conditional의 종류 https://www.slideshare.net/sbcoba/2016-deep-dive-into-spring-boot-autoconfiguration-61584342
// H2ConsoleAutoConfiguration.class
@Configuration(
proxyBeanMethods = false
)
@ConditionalOnWebApplication(
type = Type.SERVLET
)
@ConditionalOnClass({WebServlet.class}) // POM.XML에
@ConditionalOnProperty(
prefix = "spring.h2.console",
name = {"enabled"},
havingValue = "true",
matchIfMissing = false
)
@AutoConfigureAfter({DataSourceAutoConfiguration.class})
@EnableConfigurationProperties({H2ConsoleProperties.class})
public class H2ConsoleAutoConfiguration {
...
}
@Configuration 어노테이션도 @Component를 포함하기 때문에 해당 클래스도 빈으로 등록
ex) @ConditionalOnClass({WebServlet.class}) WebServlet.class는 h2 라이브러리에 포함되있음. 프로젝트 pom.xml에서 의존성 추가 전에는 WebServlet.class가 없어서 빈으로 등록하지 않는다. (IDE에서 마우스 가져다 대면 파일을 찾을 수 없다고 뜬다.) 마찬가지로 @ConditionalOnProperty()에 의해 환경 변수의 존재 유무에 따라 등록한다.
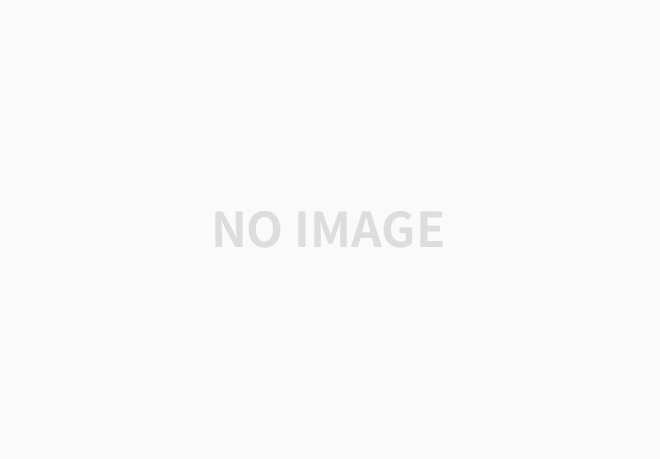
https://www.slideshare.net/sbcoba/2016-deep-dive-into-spring-boot-autoconfiguration-61584342
→ 스프링 부트는 의존성과 환경 변수를 추가하면 자동으로 설정해준다. Auto Configuration
Springboot MVC 설정 제어
기본적으로 springboot가 spring MVC를 자동으로 구성한다. 즉, 스프링 레거시 프로젝트에서 설정을 위해 작성했던 web.xml, servlet-context.xml 이나 java config 파일(WebApplicationInitializer, @EnableWebMvc)을 만들지 않아도 된다. 그렇지만 다음의 방법으로 필요에 따라 설정을 제어할 수 있다.
-
application.properties : springboot 기본 설정 커스터마이징
server.port=8080 spring.mvc.view.prefix=/WEB-INF/jsp/ spring.mvc.view.suffix=.jsp
-
@Configuration + implements WebMvcConfigurer : springboot 기본설정 + 확장 설정
interceptor, formatter, viewResolver 등을 설정
https://galid1.tistory.com/549?category=783055 포매터 등록
@Configuration public class WebConfig implements WebMvcConfigurer { @Override public void addFormatters(FormatterRegistry registry) { } @Override public void addInterceptors(InterceptorRegistry registry) { } @Override public void configureViewResolvers(ViewResolverRegistry registry) { } }
** @EnableWebMvc : 스프링 레거시 프로젝트에서 java config를 위해 사용하는 어노테이션. 핸들러 매핑, 어뎁터 등 mvc에 필요한 빈들을 자동으로 등록해줌
→ 선언하면 springboot에서 제공하는 자동 설정을 이용할 수 없다. 따라서 @Configuration + @EnableWebMvc + implements WebMvcConfigurer 로 설정. (거의 사용하지 않는 방식)
참고 springboot는 WebMvcAutoConfiguration으로 mvc 자동 설정을 한다. @EnableWebMvc를 사용하면 스프링부트가 제공하는 설정을 이용할 수 없다. @EnableWebMvc가 WebMvcConfigurationSupport를 상속 받은 DelegatingWebMvcConfiguration를 import하는데 WebMvcAutoConfiguration의 @ConditionalOnMissingBean({WebMvcConfigurationSupport.class}) 에 의하여 WebMvcAutoConfiguration이 빈으로 등록되지 않기 때문이다.
WebMvcConfigurationSupport의 spring mvc 구성을 불러옴
@ConditionalOnClass({Servlet.class, DispatcherServlet.class, WebMvcConfigurer.class})
**@ConditionalOnMissingBean({WebMvcConfigurationSupport.class})**
public class WebMvcAutoConfiguration {
}
다음 포스팅은 springboot에서 mybatis를 사용하는 법에 대해서 다룰 예정이다.
댓글